Inverse Kinematics
At the end of the sixth project I started delving into inverse kinematics and managed to implement a prototype of a FABRIK solver for IK chains. In the seventh project I implemented it properly and even implemented Foot IKs and pole vectors.
FABRIK Solver
This takes a number of joints and tries to put the last bone at a specific position without moving the first bone and only rotating the bones.
I struggled a lot with trying to implement this in the beginning since this was my first time working with bones individually, but managed to solve it pretty quickly after I got the hang of how to manipulate the bones.
Later I refactored animations to no longer store transform matrices and instead store vectors and quaternions, this made working with individual bones a lot easier and a lot stabler.
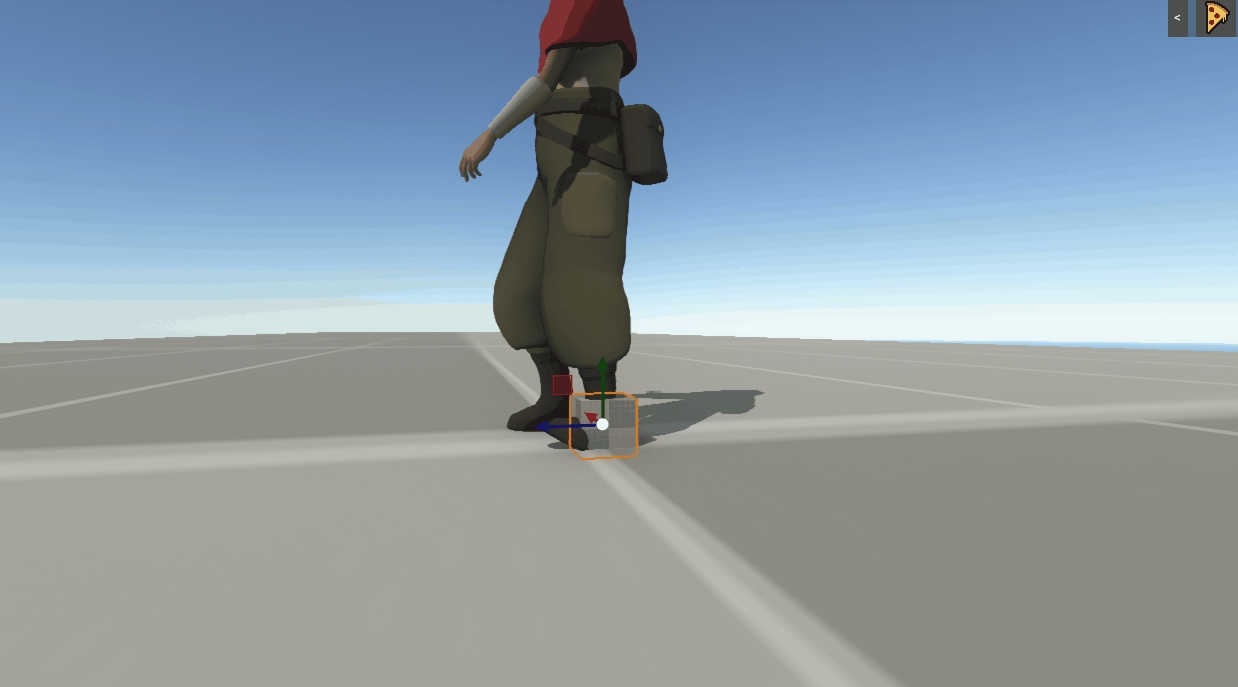
The FABRIK solver in itself is very simple to understand. You first calculate all bones world positions, then you place the Effector on the target and rotate it toward it’s parent then place the parent accordingly and so on. You then offset the whole chain to have the base joint on it’s start position. You repeat this multiple times to make it reach all the way.
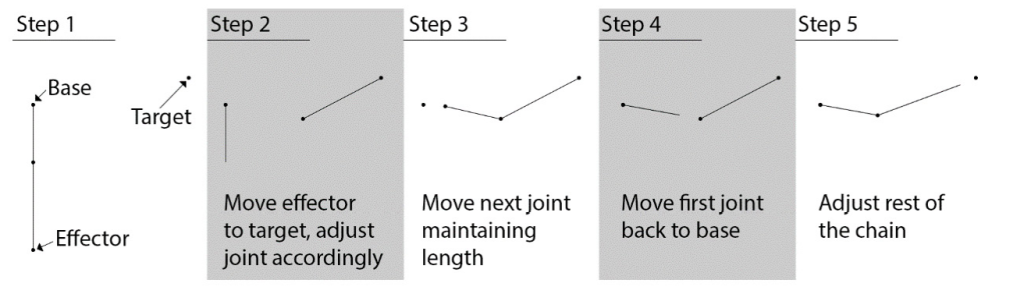
Picture from: Hands-On C++ Game Animation Programming by Gabor Szauer
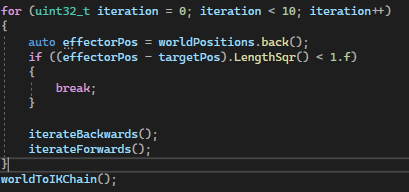
This is my implementation of the FABRIK algorithm.
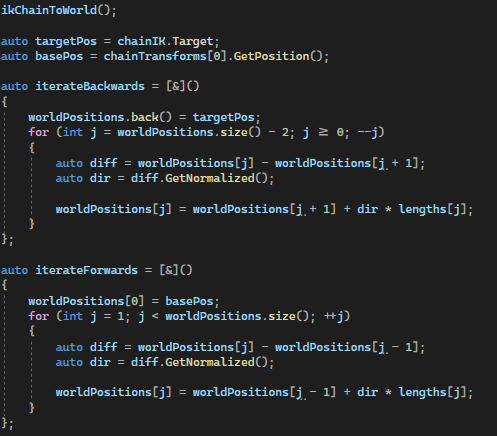
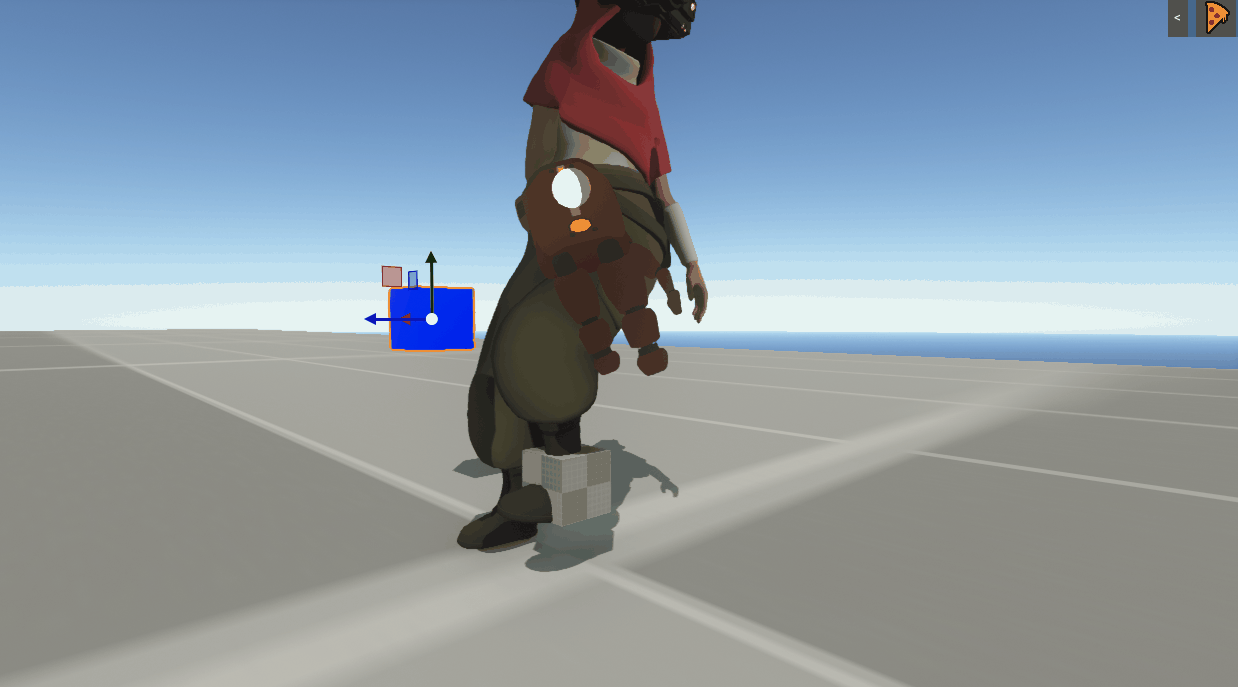
Pole Vector
A direction the chain of bones should bend in, this is a concept that only works on chains of three bones.
This was a concept I didn’t know about until one of the educators at TGA suggested it to me. Since I didn’t know what it was I had to research a fair bit to figure out what they were and how they worked.
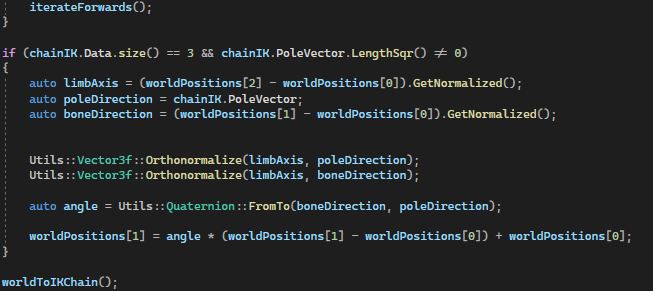
After completing the whole FABRIK solver algorithm, I adjust the knee position to be in the direction of the pole vector.
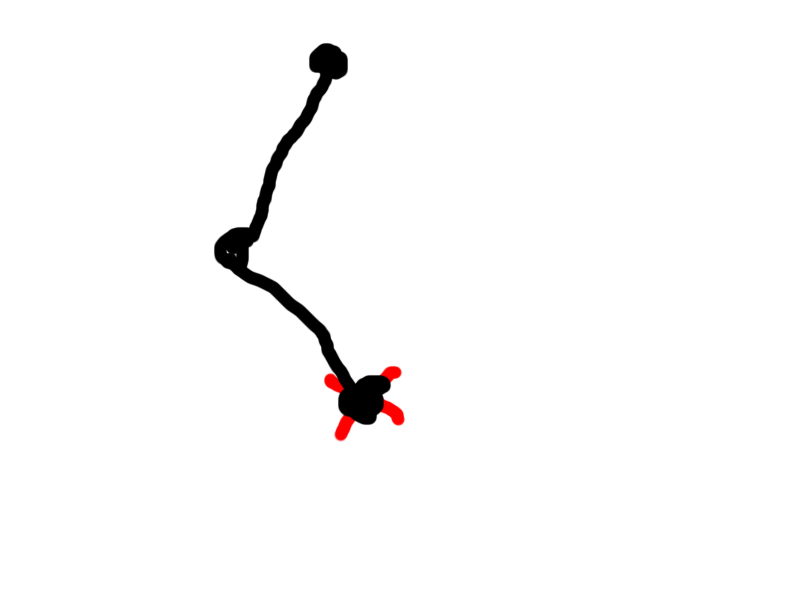
Ponder the situation in the gif. We have a IK target in red and we have completed the IK iterations, but the leg is bent the wrong way.

So we add a pole vector or as in the picture poleDirection. The boneDirection is the vector from the hip to the knee, the limbAxis is the vector from the hip to the foot.

We transform both the poleDirection and the boneDirection to be orthogonal to the limbAxis. Then we find how much we need to rotate boneDirection to make it the same as poleDirection. We then rotate the knee bone with that quaternion.
Foot Solver
Makes sure the feet of the character stays on the ground even on uneven terrain.
This is accomplished by raycasting downwards from above the foot to find where to place the foot. After we know where the foot should be, we use a IK solver and a pole vector to place the foot where it should be.
But this can feel quite jerky, so I made it more smooth by lerping toward the desired position instead.
A problem you encounter while implementing this is when the character stands on a ledge with one foot floating in the air and could reach the ground if the other leg bends.
I solved this by moving the mesh down enough for the leg to touch the ground so that the grounded leg bends instead.
Another thing to account for is to preserve the animation that the animators have made, this is done by offsetting the IK target with the same y distance as the animation says.
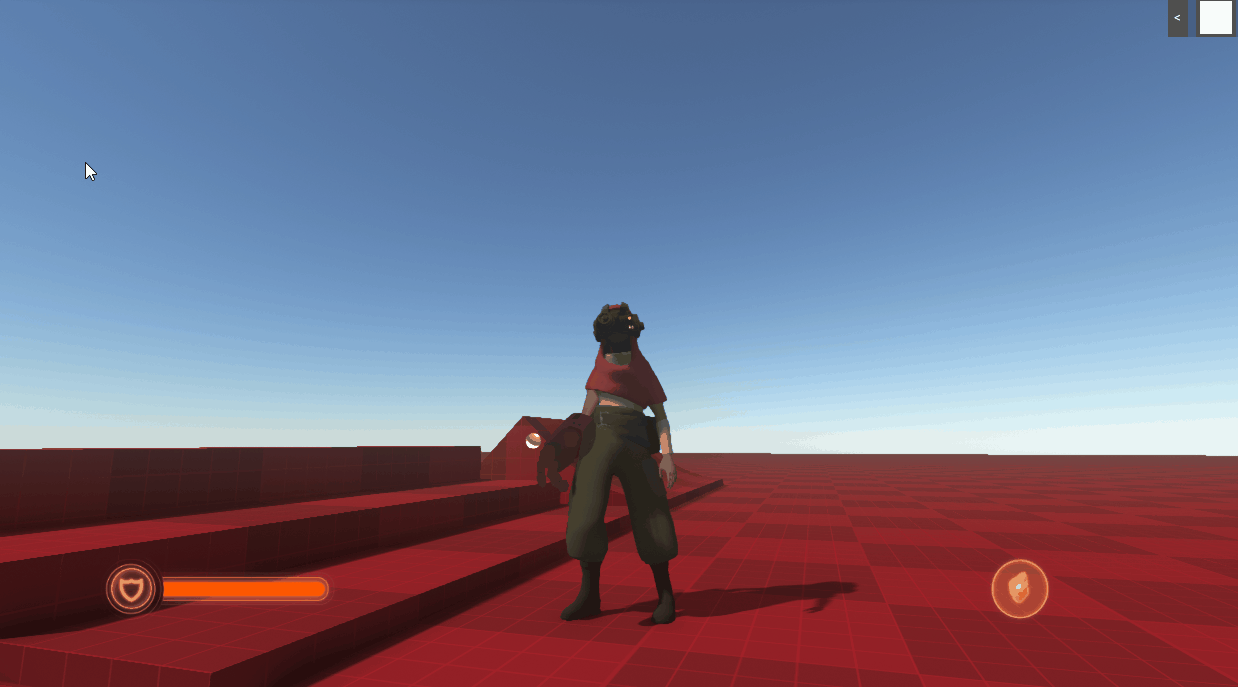

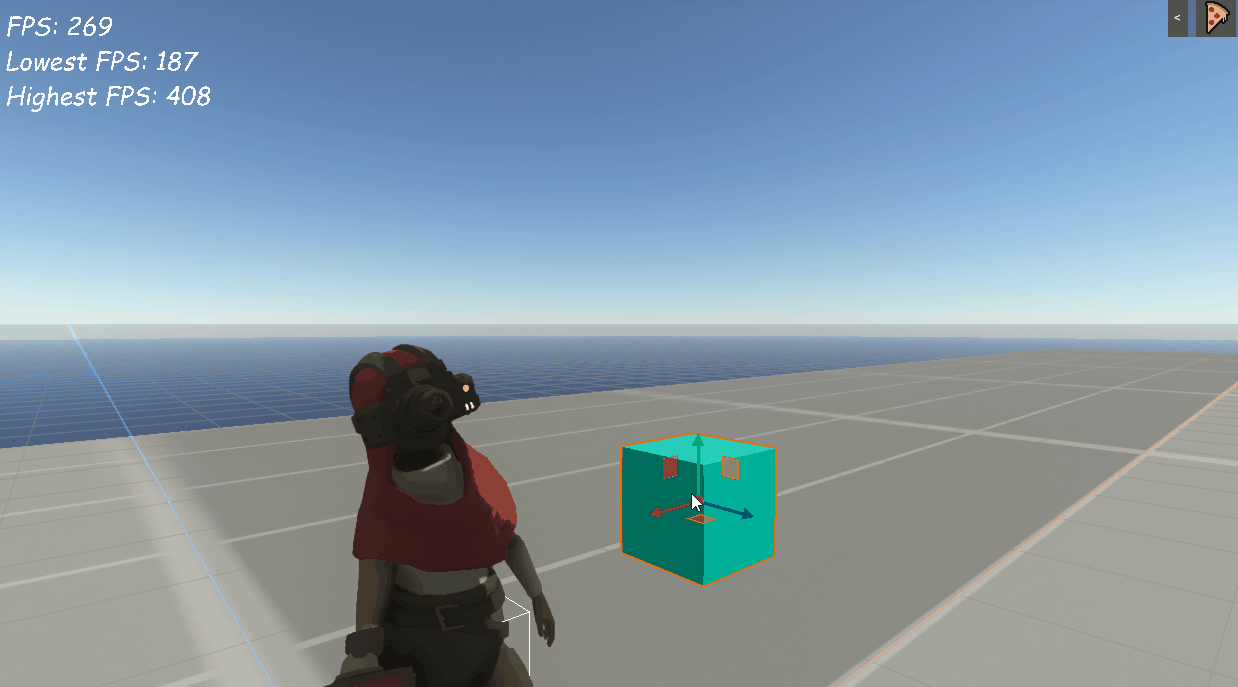
Aim IK
Rotates a bone to make it point in the direction of a target.

This is achieved by taking the bone in question into world space, then finding the desired direction.
After that, all we have to do is find how much we need to rotate the current direction to make it match the desired one, then apply.
Aim Down Sight
Using all the previously mentioned features, I made it so when the player aims, the hand and head points toward the shoot target.
The left hand also uses a FABRIK solver to stay at the bottom of the right hand to hold it up.
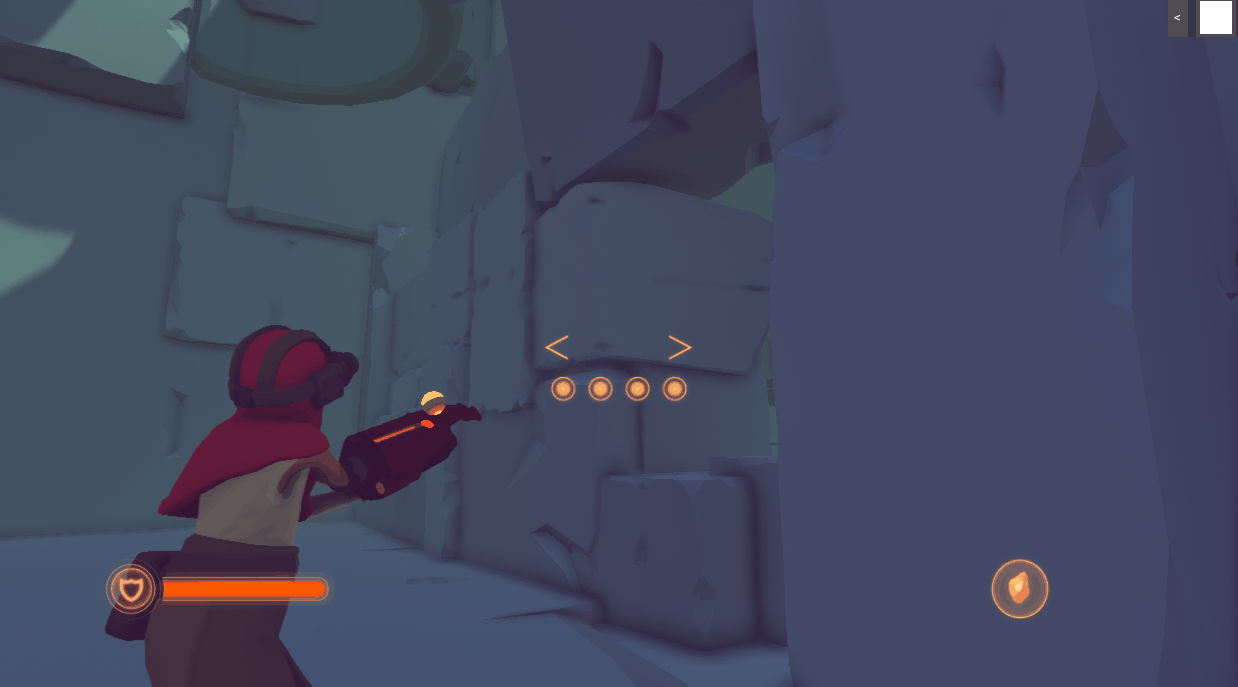